Serialize Java Object To Xml
- Java Serialize Object To Xml File
- Java Serializable Object To Xml
- C# Serialize To Xml
- Serialize Java Object To Xml String
- Java Serialize Object To File
Yes, yes I know that lots of questions were asked about this topic. But I still cannot find the solution to my problem. I have a property annotated Java object. For example Customer, like in this example. And I want a String representation of it. Google reccomends using JAXB for such purposes. But in all examples created XML file is printed to file or console, like this:
But I have to use this object and send over network in XML format. So I want to get a String which represents XML.
If you're talking about automatic XML serialization of objects, check out Castor: Castor is an Open Source data binding framework for Javatm. It's the shortest path between Java objects, XML documents and relational tables. Castor provides Java-to-XML binding, Java-to-SQL persistence, and more. Using Simple for XML serialization. Really does make it simple to go from Java objects to XML. From the developerWorks archives. Date archived: April 18, 2019 First published: November 24, 2009. Java™ developers have a variety of choices when it comes to serializing and deserializing Extensible Markup Language (XML) objects. There are quite a few interesting benefits to using XStream to serialize and deserialize XML: Configured properly, it produces very clean XML Provides significant opportunities for customization of the XML output Support for object graphs, including circular references For most use cases, the XStream instance is thread-safe. In consequence XML deserialization does not work and your application may crash on start. If the file is not huge, I suggest first serialize object to MemoryStream then write the stream to the File. This case is especially important if there is some complicated custom serialization. You can never test all cases.
How can I do this?
BobBob11 Answers
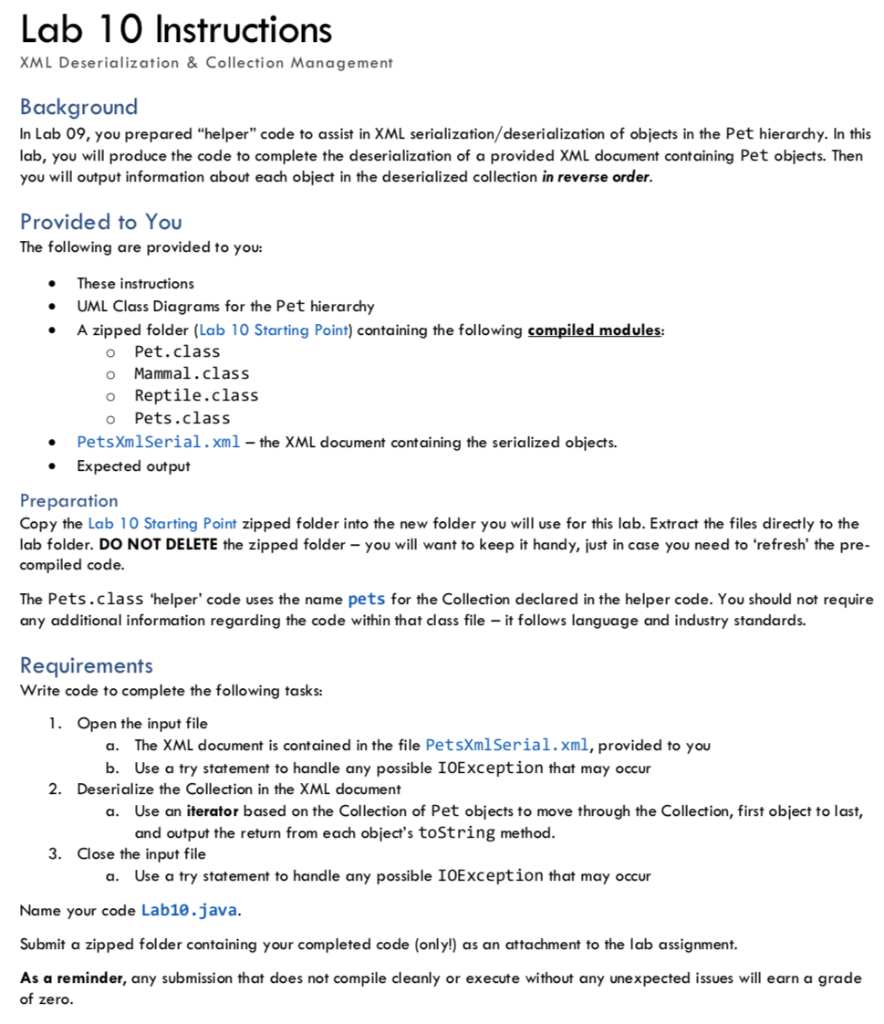
You can use the Marshaler's method for marshaling which takes a Writer as parameter:
and pass it an Implementation which can build a String object
Direct Known Subclasses: BufferedWriter, CharArrayWriter, FilterWriter, OutputStreamWriter, PipedWriter, PrintWriter, StringWriter
Call its toString method to get the actual String value.
So doing:
A4LA4LA convenient option is to use javax.xml.bind.JAXB:
The reverse process (unmarshal) would be:
No need to deal with checked exceptions in this approach.
juliaaanojuliaaanoJava Serialize Object To Xml File
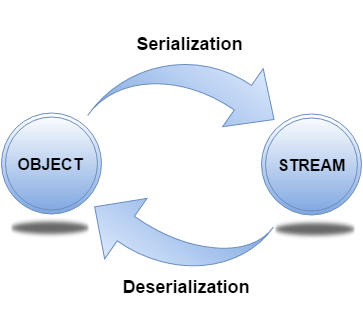
As A4L mentioning, you can use StringWriter. Providing here example code:
You can marshal it to a StringWriter
and grab its string. from toString()
.
To convert an Object to XML in Java
Java Serializable Object To Xml
Customer.java
ConvertObjToXML.java
Try with this example..
Testing And working Java code to convert java object to XML:
C# Serialize To Xml
Customer.java
createXML.java
object --> is Java class to convert it to XML
name --> is just name space like thing - for differentiate
I took the JAXB.marshal implementation and added jaxb.fragment=true to remove the XML prolog. This method can handle objects even without the XmlRootElement annotation. This also throws the unchecked DataBindingException.
If the compiler warning bothers you, here's the templated, two parameter version.
Bienvenido DavidBienvenido DavidSerialize Java Object To Xml String
Java Serialize Object To File
Use this function to convert Object to xml string (should be called as convertToXml(sourceObject, Object.class); )-->
Use this function to convert xml string to Object back -->(should be called as createObjectFromXmlString(xmlString, Object.class)
)